20. Functions and Variables¶
JMeter functions are special values that can populate fields of any Sampler or other element in a test tree. A function call looks like this:
${__functionName(var1,var2,var3)}
Where "__functionName" matches the name of a function.
Parentheses surround the parameters sent to the function, for example ${__time(YMD)}
The actual parameters vary from function to function.
Functions that require no parameters can leave off the parentheses, for example ${__threadNum}.
If a function parameter contains a comma, then be sure to escape this with "\", otherwise JMeter will treat it as a parameter delimiter. For example:
${__time(EEE\, d MMM yyyy)}If the comma is not escaped - e.g. ${__javaScript(Math.max(2,5))} - you will get an error such as:
ERROR - jmeter.functions.JavaScript: Error processing Javascript: [Math.max(2] org.mozilla.javascript.EvaluatorException: missing ) after argument list (<cmd>#1)This is because the string "Math.max(2,5)" is treated as being two parameters to the __javascript function:
Math.max(2 and 5)
Other error messages are possible.
Variables are referenced as follows:
${VARIABLE}
If an undefined function or variable is referenced, JMeter does not report/log an error - the reference is returned unchanged. For example if UNDEF is not defined as a variable, then the value of ${UNDEF} is ${UNDEF}. Variables, functions (and properties) are all case-sensitive. JMeter trims spaces from variable names before use, so for example ${__Random(1,63, LOTTERY )} will use the variable 'LOTTERY' rather than ' LOTTERY '.
Alternatively, just use / instead for the path separator - e.g. C:/test/${test} - Windows JVMs will convert the separators as necessary.
List of functions, loosely grouped into types.
Type of function | Name | Comment | Since |
---|---|---|---|
Information | threadNum | get thread number | 1.X |
Information | threadGroupName | get thread group name | 4.1 |
Information | samplerName | get the sampler name (label) | 2.5 |
Information | machineIP | get the local machine IP address | 2.6 |
Information | machineName | get the local machine name | 1.X |
Information | time | return current time in various formats | 2.2 |
Information | timeShift | return a date in various formats with the specified amount of seconds/minutes/hours/days added | 3.3 |
Information | log | log (or display) a message (and return the value) | 2.2 |
Information | logn | log (or display) a message (empty return value) | 2.2 |
Input | StringFromFile | read a line from a file | 1.9 |
Input | FileToString | read an entire file | 2.4 |
Input | CSVRead | read from CSV delimited file | 1.9 |
Input | XPath | Use an XPath expression to read from a file | 2.0.3 |
Input | StringToFile | write a string to a file | 5.2 |
Calculation | counter | generate an incrementing number | 1.X |
Formatting | dateTimeConvert | Convert a date or time from source to target format | 4.0 |
Calculation | digest | Generate a digest (SHA-1, SHA-256, MD5...) | 4.0 |
Calculation | intSum | add int numbers | 1.8.1 |
Calculation | longSum | add long numbers | 2.3.2 |
Calculation | Random | generate a random number | 1.9 |
Calculation | RandomDate | generate random date within a specific date range | 3.3 |
Calculation | RandomFromMultipleVars | extracts an element from the values of a set of variables separated by | | 3.1 |
Calculation | RandomString | generate a random string | 2.6 |
Calculation | UUID | generate a random type 4 UUID | 2.9 |
Scripting | groovy | run an Apache Groovy script | 3.1 |
Scripting | BeanShell | run a BeanShell script | 1.X |
Scripting | javaScript | process JavaScript (Nashorn) | 1.9 |
Scripting | jexl2 | evaluate a Commons Jexl2 expression | jexl2(2.1.1) |
Scripting | jexl3 | evaluate a Commons Jexl3 expression | jexl3 (3.0) |
Properties | isPropDefined | Test if a property exists | 4.0 |
Properties | property | read a property | 2.0 |
Properties | P | read a property (shorthand method) | 2.0 |
Properties | setProperty | set a JMeter property | 2.1 |
Variables | split | Split a string into variables | 2.0.2 |
Variables | eval | evaluate a variable expression | 2.3.1 |
Variables | evalVar | evaluate an expression stored in a variable | 2.3.1 |
Properties | isVarDefined | Test if a variable exists | 4.0 |
Variables | V | evaluate a variable name | 2.3RC3 |
String | char | generate Unicode char values from a list of numbers | 2.3.3 |
String | changeCase | Change case following different modes | 4.0 |
String | escapeHtml | Encode strings using HTML encoding | 2.3.3 |
String | escapeOroRegexpChars | quote meta chars used by ORO regular expression | 2.9 |
String | escapeXml | Encode strings using XMl encoding | 3.2 |
String | regexFunction | parse previous response using a regular expression | 1.X |
String | unescape | Process strings containing Java escapes (e.g. \n & \t) | 2.3.3 |
String | unescapeHtml | Decode HTML-encoded strings | 2.3.3 |
String | urldecode | Decode a application/x-www-form-urlencoded string | 2.10 |
String | urlencode | Encode a string to a application/x-www-form-urlencoded string | 2.10 |
String | TestPlanName | Return name of current test plan | 2.6 |
20.1 What can functions do¶
There are two kinds of functions: user-defined static values (or variables), and built-in functions.
User-defined static values allow the user to define variables to be replaced with their static value when
a test tree is compiled and submitted to be run. This replacement happens once at the beginning of the test
run. This could be used to replace the DOMAIN field of all HTTP requests, for example - making it a simple
matter to change a test to target a different server with the same test.
Note that variables cannot currently be nested; i.e. ${Var${N}} does not work. The __V (variable) function can be used to do this: ${__V(Var${N})}. You can also use ${__BeanShell(vars.get("Var${N}")}.
This type of replacement is possible without functions, but was less convenient and less intuitive. It required users to create default config elements that would fill in blank values of Samplers. Variables allow one to replace only part of any given value, not just filling in blank values.
With built-in functions users can compute new values at run-time based on previous response data, which thread the function is in, the time, and many other sources. These values are generated fresh for every request throughout the course of the test.
20.2 Where can functions and variables be used?¶
Functions and variables can be written into any field of any test component (apart from the TestPlan - see below). Some fields do not allow random strings because they are expecting numbers, and thus will not accept a function. However, most fields will allow functions.
Functions which are used on the Test Plan have some restrictions. JMeter thread variables will have not been fully set up when the functions are processed, so variable names passed as parameters will not be set up, and variable references will not work, so split() and regex() and the variable evaluation functions won't work. The threadNum() function won't work (and does not make sense at test plan level). The following functions should work OK on the test plan:
- intSum
- longSum
- machineName
- BeanShell
- groovy
- javaScript
- jexl2/jexl3
- random
- time
- property functions
- log functions
Configuration elements are processed by a separate thread. Therefore functions such as __threadNum do not work properly in elements such as User Defined Variables. Also note that variables defined in a UDV element are not available until the element has been processed.
SELECT item from table where name='${VAR}'not
SELECT item from table where name=${VAR}(unless VAR itself contains the quotes)
20.3 How to reference variables and functions¶
Referencing a variable in a test element is done by bracketing the variable name with '${' and '}'.
Functions are referenced in the same manner, but by convention, the names of functions begin with "__" to avoid conflict with user value names*. Some functions take arguments to configure them, and these go in parentheses, comma-delimited. If the function takes no arguments, the parentheses can be omitted.
Argument values that themselves contain commas should be escaped as necessary. If you need to include a comma in your parameter value, escape it like so: '\,'. This applies for example to the scripting functions - Javascript, Beanshell, Jexl, groovy - where it is necessary to escape any commas that may be needed in script method calls - e.g.
${__BeanShell(vars.put("name"\,"value"))}
Alternatively, you can define your script as a variable, e.g. on the Test Plan:
SCRIPT vars.put("name","value")The script can then be referenced as follows:
${__BeanShell(${SCRIPT})}There is no need to escape commas in the SCRIPT variable because the function call is parsed before the variable is replaced with its value. This works well in conjunction with the JSR223 or BeanShell Samplers, as these can be used to test Javascript, Jexl and BeanShell scripts.
Functions can reference variables and other functions, for example ${__XPath(${__P(xpath.file),${XPATH})} will use the property "xpath.file" as the file name and the contents of the variable XPATH as the expression to search for.
JMeter provides a tool to help you construct function calls for various built-in functions, which you can then copy-paste. It will not automatically escape values for you, since functions can be parameters to other functions, and you should only escape values you intend as literal.
The value of a variable or function can be reported using the __logn() function. The __logn() function reference can be used anywhere in the test plan after the variable has been defined. Alternatively, the Java Request sampler can be used to create a sample containing variable references; the output will be shown in the appropriate Listener. Note there is a Debug Sampler that can be used to display the values of variables etc. in the Tree View Listener.
20.4 The Function Helper Dialog¶
The Function Helper dialog is available from JMeter's Tools menu.
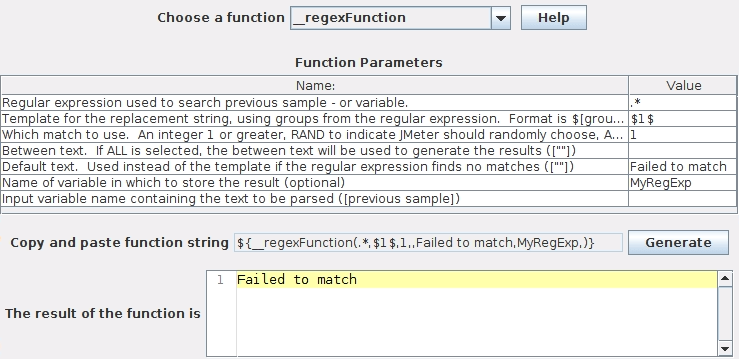
Using the Function Helper, you can select a function from the pull down, and assign values for its arguments. The left column in the table provides a brief description of the argument, and the right column is where you write in the value for that argument. Different functions take different arguments.
Once you have done this, click the "generate" button, and the appropriate string is generated for you to copy-paste into your test plan wherever you like.
20.5 Functions¶
__regexFunction¶
The Regex Function is used to parse the previous response (or the value of a variable) using any regular expression (provided by user). The function returns the template string with variable values filled in.
The __regexFunction can also store values for future use. In the sixth parameter, you can specify a reference name. After this function executes, the same values can be retrieved at later times using the syntax for user-defined values. For instance, if you enter "refName" as the sixth parameter you will be able to use:
- ${refName} to refer to the computed result of the second parameter ("Template for the replacement string") parsed by this function
- ${refName_g0} to refer to the entire match parsed by this function.
- ${refName_g1} to refer to the first group parsed by this function.
- ${refName_g#} to refer to the nth group parsed by this function.
- ${refName_matchNr} to refer to the number of groups found by this function.
Parameters ¶
- An integer - Tells JMeter to use that match. '1' for the first found match, '2' for the second, and so on
- RAND - Tells JMeter to choose a match at random.
- ALL - Tells JMeter to use all matches, and create a template string for each one and then append them all together. This option is little used.
- A float number between 0 and 1 - tells JMeter to find the Xth match using the formula: (number_of_matches_found * float_number) rounded to nearest integer.
Stored values are ${refName} (the replacement template string) and ${refName_g#} where "#" is the group number from the regular expression ("0" can be used to refer to the entire match).
__counter¶
The counter generates a new number each time it is called, starting with 1 and incrementing by +1 each time. The counter can be configured to keep each simulated user's values separate, or to use the same counter for all users. If each user's values is incremented separately, that is like counting the number of iterations through the test plan. A global counter is like counting how many times that request was run.
The counter uses an integer variable to hold the count, which therefore has a maximum of 2,147,483,647.
The counter function instances are completely independent. The global counter - "FALSE" - is separately maintained by each counter instance.
Multiple __counter function calls in the same iteration won't increment the value further.
If you want to have a count that increments for each sample, use the function in a Pre-Processor such as User Parameters.
Parameters ¶
Stored values are of the form ${refName}. This allows you to keep one counter and refer to its value in multiple places.
__threadNum¶
The thread number function simply returns the number of the thread currently being executed. These numbers are only locally unique with respect to their ThreadGroup, meaning thread #1 in one threadgroup is indistinguishable from thread #1 in another threadgroup, from the point of view of this function.
ctx.getThreadNum()
There are no arguments for this function.
Usage Example:
${__threadNum}returns a number between 1 and the max number of running threads configured in the containing Thread Group
__threadGroupName¶
The thread group name function simply returns the name of the thread group being executed.
There are no arguments for this function.
Usage Example:
${__threadGroupName}
__intSum¶
The intSum function can be used to compute the sum of two or more integer values.
Parameters ¶
Examples:
${__intSum(2,5,MYVAR)}will return 7 (2+5) and store the result in MYVAR variable. So ${MYVAR} will be equal to 7.
${__intSum(2,5,7)}will return 14 (2+5+7) and store the result in MYVAR variable.
${__intSum(1,2,5,${MYVAR})}will return 16 if MYVAR value is equal to 8, 1+2+5+${MYVAR})
__longSum¶
The longSum function can be used to compute the sum of two or more long values, use this instead of __intSum whenever you know your values will not be in the interval -2147483648 to 2147483647.
Parameters ¶
Examples:
${__longSum(2,5,MYVAR)}will return 7 (2+5) and store the result in MYVAR variable. So ${MYVAR} will be equal to 7.
${__longSum(2,5,7)}will return 14 (2+5+7) and store the result in MYVAR variable.
${__longSum(1,2,5,${MYVAR})}will return 16 if MYVAR value is equal to 8, 1+2+5+${MYVAR})
__StringFromFile¶
The StringFromFile function can be used to read strings from a text file. This is useful for running tests that require lots of variable data. For example when testing a banking application, 100s or 1000s of different account numbers might be required.
See also the CSV Data Set Config test element which may be easier to use. However, that does not currently support multiple input files.
Each time it is called it reads the next line from the file. All threads share the same instance, so different threads will get different lines. When the end of the file is reached, it will start reading again from the beginning, unless the maximum loop count has been reached. If there are multiple references to the function in a test script, each will open the file independently, even if the file names are the same. [If the value is to be used again elsewhere, use different variable names for each function call.]
If an error occurs opening or reading the file, then the function returns the string "**ERR**"
Parameters ¶
The file name parameter is resolved when the file is opened or re-opened.
The reference name parameter (if supplied) is resolved every time the function is executed.
Using sequence numbers:
When using the optional sequence numbers, the path name is used as the format string for java.text.DecimalFormat. The current sequence number is passed in as the only parameter. If the optional start number is not specified, the path name is used as is. Useful formatting sequences are:
- #
- insert the number, with no leading zeros or spaces
- 000
- insert the number packed out to three digits with leading zeros if necessary
- pin#'.'dat
-
Will generate the digits without leading zeros and treat the dot literally like
pin1.dat, …, pin9.dat, pin10.dat, …, pin9999.dat - pin000'.'dat
-
Will generate leading zeros while keeping the dot. When the numbers start having more digits
then those three digits that this format suggests, the sequence will use more digits as can be seen in
pin001.dat, … pin099.dat, …, pin999.dat, …, pin9999.dat - pin'.'dat#
-
Will append digits without leading zeros while keeping the dot and generate
pin.dat1, …, pin.dat9, …, pin.dat999
If more digits are required than there are formatting characters, the number will be
expanded as necessary.
To prevent a formatting character from being interpreted,
enclose it in single quotes. Note that "." is a formatting character,
and must be enclosed in single quotes
(though #. and 000. work as expected in locales where the decimal point is also ".")
In other locales (e.g. fr), the decimal point is "," - which means that "#."
becomes "nnn,".
See the documentation for DecimalFormat for full details.
If the path name does not contain any special formatting characters,
the current sequence number will be appended to the name, otherwise
the number will be inserted according to the formatting instructions.
If the start sequence number is omitted, and the end sequence number is specified,
the sequence number is interpreted as a loop count, and the file will be used at most "end" times.
In this case the filename is not formatted.
${__StringFromFile(PIN#'.'DAT,,1,2)} - reads PIN1.DAT, PIN2.DAT
${__StringFromFile(PIN.DAT,,,2)} - reads PIN.DAT twice
Note that the "." in PIN.DAT above should not be quoted.
In this case the start number is omitted, so the file name is used exactly as is.
__machineName¶
The machineName function returns the local host name. This uses the Java method InetAddress.getLocalHost() and passes it to getHostName()
Parameters ¶
Examples:
${__machineName()}will return the host name of the machine
${__machineName}will return the host name of the machine
__machineIP¶
The machineIP function returns the local IP address. This uses the Java method InetAddress.getLocalHost() and passes it to getHostAddress()
Parameters ¶
Examples:
${__machineIP()}will return the IP address of the machine
${__machineIP}will return the IP address of the machine
__javaScript¶
The javaScript function executes a piece of JavaScript (not Java!) code and returns its value
The JMeter Javascript function calls a standalone JavaScript interpreter. Javascript is used as a scripting language, so you can do calculations etc.
For Rhino engine, please see Mozilla Rhino Overview
The following variables are made available to the script:
- log - the Logger for the function
- ctx - JMeterContext object
- vars - JMeterVariables object
- threadName - String containing the current thread name
- sampler - current Sampler object (if any)
- sampleResult - previous SampleResult object (if any)
- props - JMeterProperties (class java.util.Properties) object
Rhinoscript allows access to static methods via its Packages object. See the Scripting Java documentation. For example one can access the JMeterContextService static methods thus: Java.type("org.apache.jmeter.threads.JMeterContextService").getTotalThreads()
Parameters ¶
- new Date() - return the current date and time
- Math.floor(Math.random()*(${maxRandom}+1)) - a random number between 0 and the variable maxRandom
- ${minRandom}+Math.floor(Math.random()*(${maxRandom}-${minRandom}+1)) - a random number between the variables minRandom and maxRandom
- "${VAR}"=="abcd"
${__javaScript('${sp}'.slice(7\,99999))}the comma after 7 is escaped.
Examples:
${__javaScript(new Date())}will return Sat Jan 09 2016 16:22:15 GMT+0100 (CET)
${__javaScript(new Date(),MYDATE)}will return Sat Jan 09 2016 16:22:15 GMT+0100 (CET) and store it under variable MYDATE
${__javaScript(Math.floor(Math.random()*(${maxRandom}+1)),MYRESULT)}will use maxRandom variable, return a random value between 0 and maxRandom and store it in MYRESULT
${__javaScript(${minRandom}+Math.floor(Math.random()*(${maxRandom}-${minRandom}+1)),MYRESULT)}will use maxRandom and minRandom variables, return a random value between maxRandom and minRandom and store it under variable MYRESULT
${__javaScript("${VAR}"=="abcd",MYRESULT)}will compare the value of VAR variable with abcd, return true or false and store the result in MYRESULT
__Random¶
The random function returns a random number that lies between the given min and max values.
Parameters ¶
Examples:
${__Random(0,10)}will return a random number between 0 and 10
${__Random(0,10, MYVAR)}will return a random number between 0 and 10 and store it in MYVAR. ${MYVAR} will contain the random number
__RandomDate¶
The RandomDate function returns a random date that lies between the given start date and end date values.
Parameters ¶
Examples:
${__RandomDate(,,2050-07-08,,)}will return a random date between now and 2050-07-08. For example 2039-06-21
${__RandomDate(dd MM yyyy,,08 07 2050,,)}will return a random date with a custom format like 04 03 2034
__RandomString¶
The RandomString function returns a random String of length using characters in chars to use.
Parameters ¶
Examples:
${__RandomString(5)}will return a random string of 5 characters which can be readable or not
${__RandomString(10,abcdefg)}will return a random string of 10 characters picked from abcdefg set, like cdbgdbeebd or adbfeggfad, …
${__RandomString(6,a12zeczclk, MYVAR)}will return a random string of 6 characters picked from a12zeczclk set and store the result in MYVAR, MYVAR will contain string like 2z22ak or z11kce, …
__RandomFromMultipleVars¶
The RandomFromMultipleVars function returns a random value based on the variable values provided by Source Variables.
The variables can be simple or multi-valued as they can be generated by the following extractors:- Boundary Extractor
- Regular Expression Extractor
- CSS Selector Extractor
- JSON Extractor
- XPath Extractor
- XPath2 Extractor
Parameters ¶
Examples:
${__RandomFromMultipleVars(val)}will return a random string based on content of variable val taking into account whether they are multi-value or not
${__RandomFromMultipleVars(val1|val2)}will return a random string based on content of variables val1 and val2 taking into account whether they are multi-value or not
${__RandomFromMultipleVars(val1|val2, MYVAR)}will return a random string based on content of variables val1 and val2 taking into account whether they are multi-value or not and store the result in MYVAR
__UUID¶
The UUID function returns a pseudo random type 4 Universally Unique IDentifier (UUID).
Parameters ¶
Examples:
${__UUID()}will return UUIDs with this format : c69e0dd1-ac6b-4f2b-8d59-5d4e8743eecd
__CSVRead¶
The CSVRead function returns a string from a CSV file (c.f. StringFromFile)
NOTE: JMeter supports multiple file names.
In most cases, the newer CSV Data Set Config element is easier to use.
When a filename is first encountered, the file is opened and read into an internal array. If a blank line is detected, this is treated as end of file - this allows trailing comments to be used.
All subsequent references to the same file name use the same internal array. N.B. the filename case is significant to the function, even if the OS doesn't care, so CSVRead(abc.txt,0) and CSVRead(aBc.txt,0) would refer to different internal arrays.
The *ALIAS feature allows the same file to be opened more than once, and also allows for shorter file names.
Each thread has its own internal pointer to its current row in the file array. When a thread first refers to the file it will be allocated the next free row in the array, so each thread will access a different row from all other threads. [Unless there are more threads than there are rows in the array.]
Parameters ¶
For example, you could set up some variables as follows:
- COL1a ${__CSVRead(random.txt,0)}
- COL2a ${__CSVRead(random.txt,1)}${__CSVRead(random.txt,next)}
- COL1b ${__CSVRead(random.txt,0)}
- COL2b ${__CSVRead(random.txt,1)}${__CSVRead(random.txt,next)}
__property¶
The property function returns the value of a JMeter property. If the property value cannot be found, and no default has been supplied, it returns the property name. When supplying a default value, there is no need to provide a function name - the parameter can be set to null, and it will be ignored.
For example:
- ${__property(user.dir)} - return value of user.dir
- ${__property(user.dir,UDIR)} - return value of user.dir and save in UDIR
- ${__property(abcd,ABCD,atod)} - return value of property abcd (or "atod" if not defined) and save in ABCD
- ${__property(abcd,,atod)} - return value of property abcd (or "atod" if not defined) but don't save it
Parameters ¶
__P¶
This is a simplified property function which is intended for use with properties defined on the command line. Unlike the __property function, there is no option to save the value in a variable, and if no default value is supplied, it is assumed to be 1. The value of 1 was chosen because it is valid for common test variables such as loops, thread count, ramp up etc.
For example:
Define the property value:
jmeter -Jgroup1.threads=7 -Jhostname1=www.realhost.edu
Fetch the values:
${__P(group1.threads)} - return the value of group1.threads
${__P(group1.loops)} - return the value of group1.loops
${__P(hostname,www.dummy.org)} - return value of property hostname or www.dummy.org if not defined
In the examples above, the first function call would return 7,
the second would return 1 and the last would return www.dummy.org
(unless those properties were defined elsewhere!)
Parameters ¶
__log¶
The log function logs a message, and returns its input string
Parameters ¶
The OUT and ERR log level names are used to direct the output to System.out and System.err respectively. In this case, the output is always printed - it does not depend on the current log setting.
- ${__log(Message)}
- written to the log file as " … thread Name : Message"
- ${__log(Message,OUT)}
- written to console window
- ${__log(${VAR},,,VAR=)}
- written to log file as " … thread Name VAR=value"
__logn¶
The logn function logs a message, and returns the empty string
Parameters ¶
The OUT and ERR log level names are used to direct the output to System.out and System.err respectively. In this case, the output is always printed - it does not depend on the current log setting.
- ${__logn(VAR1=${VAR1},OUT)}
- write the value of the variable to the console window
__BeanShell¶
The BeanShell function evaluates the script passed to it, and returns the result.
For full details on using BeanShell, please see the BeanShell web-site at http://www.beanshell.org/
A single instance of a function may be called from multiple threads. However the function execute() method is synchronised.
If the property "beanshell.function.init" is defined, it is passed to the Interpreter as the name of a sourced file. This can be used to define common methods and variables. There is a sample init file in the bin directory: BeanShellFunction.bshrc.
The following variables are set before the script is executed:
- log - the Logger for the BeanShell function (*)
- ctx - JMeterContext object
- vars - JMeterVariables object
- props - JMeterProperties (class java.util.Properties) object
- threadName - the threadName (String)
- Sampler - the current Sampler, if any
- SampleResult - the current SampleResult, if any
Parameters ¶
Example:
- ${__BeanShell(123*456)}
- returns 56088
- ${__BeanShell(source("function.bsh"))}
- processes the script in function.bsh
__groovy¶
The __groovy function evaluates Apache Groovy scripts passed to it, and returns the result.
If the property "groovy.utilities" is defined, it will be loaded by the ScriptEngine. This can be used to define common methods and variables. There is a sample init file in the bin directory: utility.groovy.
The following variables are set before the script is executed:
- log - the Logger for the groovy function (*)
- ctx - JMeterContext object
- vars - JMeterVariables object
- props - JMeterProperties (class java.util.Properties) object
- threadName - the threadName (String)
- sampler - the current Sampler, if any
- prev - the previous SampleResult, if any
- OUT - System.out
(*) means that this is set before the init file, if any, is processed. Other variables vary from invocation to invocation.
For instance don't do the following:
${__groovy("${myVar}".substring(0\,2))}
Imagine that the variable myVar changes with each transaction, the Groovy above cannot be cached as the script changes each time.
Instead do the following, which can be cached:
${__groovy(vars.get("myVar").substring(0\,2))}
Parameters ¶
Example:
-
${__groovy(123*456)}
- returns 56088
-
${__groovy(vars.get("myVar").substring(0\,2))}
- If var's value is JMeter, it will return JM as it runs String.substring(0,2). Note that , has been escaped to \,
__split¶
The split function splits the string passed to it according to the delimiter, and returns the original string. If any delimiters are adjacent, "?" is returned as the value. The split strings are returned in the variables ${VAR_1}, ${VAR_2} etc. The count of variables is returned in ${VAR_n}. A trailing delimiter is treated as a missing variable, and "?" is returned. Also, to allow it to work better with the ForEach controller, __split now deletes the first unused variable in case it was set by a previous split.
Example:Define VAR="a||c|" in the test plan.
${__split(${VAR},VAR,|)}
This will return the contents of VAR, i.e. "a||c|" and set the following variables:
VAR_n=4
VAR_1=a
VAR_2=?
VAR_3=c
VAR_4=?
VAR_5=null
Parameters ¶
__XPath¶
The XPath function reads an XML file and matches the XPath. Each time the function is called, the next match will be returned. At end of file, it will wrap around to the start. If no nodes matched, then the function will return the empty string, and a warning message will be written to the JMeter log file.
${__XPath(/path/to/build.xml, //target/@name)}This will match all targets in build.xml and return the contents of the next name attribute
Parameters ¶
__setProperty¶
The setProperty function sets the value of a JMeter property. The default return value from the function is the empty string, so the function call can be used anywhere functions are valid.
The original value can be returned by setting the optional 3rd parameter to "true".
Properties are global to JMeter, so can be used to communicate between threads and thread groups
Parameters ¶
__time¶
The time function returns the current time in various formats.
Parameters ¶
If the format string is omitted, then the function returns the current time in milliseconds since the epoch. If the format matches "/ddd" (where ddd are decimal digits), then the function returns the current time in milliseconds divided by the value of ddd. For example, "/1000" returns the current time in seconds since the epoch. Otherwise, the current time is passed to DateTimeFormatter. The following shorthand aliases are provided:
- YMD = yyyyMMdd
- HMS = HHmmss
- YMDHMS = yyyyMMdd-HHmmss
- USER1 = whatever is in the JMeter property time.USER1
- USER2 = whatever is in the JMeter property time.USER2
The defaults can be changed by setting the appropriate JMeter property, e.g. time.YMD=yyMMdd
${__time(dd/MM/yyyy,)}will return 21/01/2018 if ran on 21 january 2018
${__time(YMD,)}will return 20180121 if ran on 21 january 2018
${__time()}will return time in millis 1516540541624
__jexl2¶
The jexl function returns the result of evaluating a Commons JEXL expression. See links below for more information on JEXL expressions.
The __jexl2 function uses Commons JEXL 2
Parameters ¶
The following variables are made available to the script:
- log - the Logger for the function
- ctx - JMeterContext object
- vars - JMeterVariables object
- props - JMeterProperties (class java.util.Properties) object
- threadName - String containing the current thread name
- sampler - current Sampler object (if any)
- sampleResult - previous SampleResult object (if any)
- OUT - System.out - e.g. OUT.println("message")
Jexl can also create classes and call methods on them, for example:
Systemclass=log.class.forName("java.lang.System"); now=Systemclass.currentTimeMillis();Note that the Jexl documentation on the web-site wrongly suggests that "div" does integer division. In fact "div" and "/" both perform normal division. One can get the same effect as follows:
i= 5 / 2; i.intValue(); // or use i.longValue()
__jexl3¶
The jexl function returns the result of evaluating a Commons JEXL expression. See links below for more information on JEXL expressions.
The __jexl3 function uses Commons JEXL 3
Parameters ¶
The following variables are made available to the script:
- log - the Logger for the function
- ctx - JMeterContext object
- vars - JMeterVariables object
- props - JMeterProperties (class java.util.Properties) object
- threadName - String containing the current thread name
- sampler - current Sampler object (if any)
- sampleResult - previous SampleResult object (if any)
- OUT - System.out - e.g. OUT.println("message")
Jexl can also create classes and call methods on them, for example:
Systemclass=log.class.forName("java.lang.System"); now=Systemclass.currentTimeMillis();Note that the Jexl documentation on the web-site wrongly suggests that "div" does integer division. In fact "div" and "/" both perform normal division. One can get the same effect as follows:
i= 5 / 2; i.intValue(); // or use i.longValue()
__V¶
The V (variable) function returns the result of evaluating a variable name expression. This can be used to evaluate nested variable references (which are not currently supported).
For example, if one has variables A1,A2 and N=1:
- ${A1} - works OK
- ${A${N}} - does not work (nested variable reference)
- ${__V(A${N})} - works OK. A${N} becomes A1, and the __V function returns the value of A1
Parameters ¶
__evalVar¶
The evalVar function returns the result of evaluating an expression stored in a variable.
This allows one to read a string from a file, and process any variable references in it. For example, if the variable "query" contains "select ${column} from ${table}" and "column" and "table" contain "name" and "customers", then ${__evalVar(query)} will evaluate as "select name from customers".
__eval¶
The eval function returns the result of evaluating a string expression.
This allows one to interpolate variable and function references in a string which is stored in a variable. For example, given the following variables:
- name=Smith
- column=age
- table=birthdays
- SQL=select ${column} from ${table} where name='${name}'
This can be used in conjunction with CSV Dataset, for example where the both SQL statements and the values are defined in the data file.
__char¶
The char function returns the result of evaluating a list of numbers as Unicode characters. See also __unescape(), below.
This allows one to add arbitrary character values into fields.
Parameters ¶
Examples:
${__char(13,10)} = ${__char(0xD,0xA)} = ${__char(015,012)} = CRLF
${__char(165)} = ¥ (yen)
__unescape¶
The unescape function returns the result of evaluating a Java-escaped string. See also __char() above.
This allows one to add characters to fields which are otherwise tricky (or impossible) to define via the GUI.
Examples:
${__unescape(\r\n)} = CRLF
${__unescape(1\t2)} = 1[tab]2
__unescapeHtml¶
Function to unescape a string containing HTML entity escapes to a string containing the actual Unicode characters corresponding to the escapes. Supports HTML 4.0 entities.
For example, the string
${__unescapeHtml(<Français>)}will return <Français>.
If an entity is unrecognized, it is left alone, and inserted verbatim into the result string. e.g. ${__unescapeHtml(>&zzzz;x)} will return >&zzzz;x.
Uses StringEscapeUtils#unescapeHtml(String) from Commons Lang.
__escapeHtml¶
Function which escapes the characters in a String using HTML entities. Supports HTML 4.0 entities.
For example,
${__escapeHtml("bread" & "butter")}return: "bread" & "butter".
Uses StringEscapeUtils#escapeHtml(String) from Commons Lang.
__urldecode¶
Function to decode a application/x-www-form-urlencoded string. Note: use UTF-8 as the encoding scheme.
For example, the string
${__urldecode(Word+%22school%22+is+%22%C3%A9cole%22+in+french)}returns Word "school" is "école" in french.
Uses Java class URLDecoder.
Parameters ¶
__urlencode¶
Function to encode a string to a application/x-www-form-urlencoded string.
For example, the string
${__urlencode(Word "school" is "école" in french)}returns Word+%22school%22+is+%22%C3%A9cole%22+in+french.
Uses Java class URLEncoder.
Parameters ¶
__FileToString¶
The FileToString function can be used to read an entire file. Each time it is called it reads the entire file.
If an error occurs opening or reading the file, then the function returns the string "**ERR**"
Parameters ¶
The file name, encoding and reference name parameters are resolved every time the function is executed.
__samplerName¶
The samplerName function returns the name (i.e. label) of the current sampler.
The function does not work in Test elements that don't have an associated sampler. For example the Test Plan. Configuration elements also don't have an associated sampler. However some Configuration elements are referenced directly by samplers, such as the HTTP Header Manager and Http Cookie Manager, and in this case the functions are resolved in the context of the Http Sampler. Pre-Processors, Post-Processors and Assertions always have an associated Sampler.
Example:
${__samplerName()}
Parameters ¶
__TestPlanName¶
The TestPlanName function returns the name of the current test plan (can be used in Including Plans to know the name of the calling test plan).
Example:
${__TestPlanName}will return the file name of your test plan, for example if plan is in a file named Demo.jmx, it will return "Demo.jmx
__escapeOroRegexpChars¶
Function which escapes the ORO Regexp meta characters, it is the equivalent of \Q \E in Java Regexp Engine.
For example,
${__escapeOroRegexpChars([^"].+?,)}returns: \[\^\"\]\.\+\?.
Uses Perl5Compiler#quotemeta(String) from ORO.
Parameters ¶
__escapeXml¶
Function which escapes the characters in a String using XML 1.0 entities.
For example,
${__escapeXml("bread" & 'butter')}returns: "bread" & 'butter'.
Uses StringEscapeUtils#escapeXml10(String) from Commons Lang.
__timeShift¶
The timeShift function returns a date in the given format with the specified amount of seconds, minutes, hours, days or months added
Parameters ¶
- PT20.345S parses as 20.345 seconds
- PT15M parses as 15 minutes
- PT10H parses as 10 hours
- P2D parses as 2 days
- -P6H3M parses as -6 hours and -3 minutes
Examples:
${__timeShift(dd/MM/yyyy,21/01/2018,P2D,,)}returns 23/01/2018
${__timeShift(dd MMMM yyyy,21 février 2018,P2D,fr_FR,)}returns 23 février 2018
${__timeShift(,10000,PT10S,,)}returns 20000 = 10sec input + 10sec shift
${__timeShift(,,PT10S,,)}returns 1632158276770 = 1632158266770 ms (now) + 10sec shift
__digest¶
The digest function returns an encrypted value in the specific hash algorithm with the optional salt, upper case and variable name.
Parameters ¶
- MD2
- MD5
- SHA-1
- SHA-224
- SHA-256
- SHA-384
- SHA-512
Examples:
${__digest(MD5,Errare humanum est,,,)}returns c49f00b92667a35c63708933384dad52
${__digest(SHA-256,Felix qui potuit rerum cognoscere causas,mysalt,,)}returns a3bc6900fe2b2fc5fa8a601a4a84e27a079bf2c581d485009bc5c00516729ac7
__dateTimeConvert¶
The __dateTimeConvert function converts a date that is in source format to a target format storing the result optionally in the variable name.
Parameters ¶
Example:
${__dateTimeConvert(01212018,MMddyyyy,dd/MM/yyyy,)}returns 21/01/2018
With epoch time value: 1526574881000,
${__dateTimeConvert(1526574881000,,dd/MM/yyyy HH:mm,)}returns 17/05/2018 16:34 in UTC time(-Duser.timezone=GMT)
__isPropDefined¶
The __isPropDefined function returns true if property exists or false if not.
Parameters ¶
Example:
${__isPropDefined(START.HMS)}will return true
__isVarDefined¶
The __isVarDefined function returns true if variable exists or false if not.
Parameters ¶
Example:
${__isVarDefined(JMeterThread.last_sample_ok)}will return true
__changeCase¶
The change case function returns a string value which case has been changed following a specific mode. Result can optionally be saved in a JMeter variable.
Parameters ¶
- UPPER result as AB-CD EF
- LOWER result as ab-cd ed
- CAPITALIZE result as Ab-CD eF
Examples:
${__changeCase(Avaro omnia desunt\, inopi pauca\, sapienti nihil,UPPER,)}will return AVARO OMNIA DESUNT, INOPI PAUCA, SAPIENTI NIHIL
${__changeCase(LABOR OMNIA VINCIT IMPROBUS,LOWER,)}will return labor omnia vincit improbus
${__changeCase(omnibus viis romam pervenitur,CAPITALIZE,)}will return Omnibus viis romam pervenitur
__StringToFile¶
The __StringToFile function can be used to write a string to a file. Each time it is called it writes a string to file appending or overwriting.
The default return value from the function is the empty string
Parameters ¶
If you need to insert a line break in your content, use \n in your string.
20.6 Pre-defined Variables¶
Most variables are set by calling functions or by test elements such as User Defined Variables; in which case the user has full control over the variable name that is used. However some variables are defined internally by JMeter. These are listed below.
- COOKIE_cookiename - contains the cookie value (see HTTP Cookie Manager)
- JMeterThread.last_sample_ok - whether or not the last sample was OK - true/false. Note: this is updated after PostProcessors and Assertions have been run.
- START variables (see next section)
20.6 Pre-defined Properties¶
The set of JMeter properties is initialised from the system properties defined when JMeter starts; additional JMeter properties are defined in jmeter.properties, user.properties or on the command line.
Some built-in properties are defined by JMeter. These are listed below. For convenience, the START properties are also copied to variables with the same names.
- START.MS - JMeter start time in milliseconds
- START.YMD - JMeter start time as yyyyMMdd
- START.HMS - JMeter start time as HHmmss
- TESTSTART.MS - test start time in milliseconds
Please note that the START variables / properties represent JMeter startup time, not the test start time. They are mainly intended for use in file names etc.